Many Indians use wearable devices to monitor their health and fitness today. A fitness tracker is a device that tracks your orientation, movement, and rotation using sensors. The device takes data and translates it into your daily activity, calories burned, quality of sleep, and the number of steps taken.
Fitness bands have taken the market by storm because they help us keep track of the calories we burn each day. This hypothetical fitness band is an Internet of Things (IoT) project that uses heart rate to calculate calories burned and a smartphone application to assist the user in monitoring his or her activity level.
Hardware required:
- NodeMCU
- Pulse sensor(PLSNSR1)
- LED
- Resistor (220 Ohm)
- Jumper wires
How Fitness band works?
This fitness band system measures heart activity in Beats per minute using a heartbeat sensor ( BPM ). The heartbeat is a crucial indicator of physical exertion. When we engage in physical activity, our heart rate increases, and when we relax, it returns to normal. This makes heart rate an excellent indicator of the number of calories burned. In addition to heart rate, we require additional characteristics such as the user’s gender, age, and weight. These variables will allow us to calculate the calories burned for a certain time period.
When the system is powered on, NodeMCU awaits start instructions from the Blynk app, which is connected to NodeMCU’s local network via Wi-Fi. The user must then enter personal profile information including gender, age, and weight. The user can then turn ON the fitness band using the “Device ON” button within the Blynk app. Now NodeMCU will begin reading analog heartbeat data and will also keep track of the time.
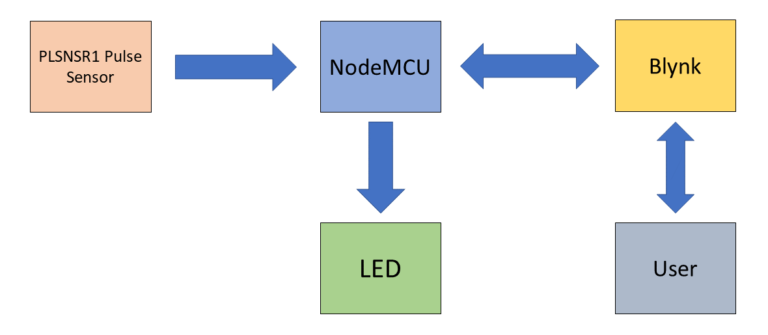
This fitness bracelet measures heart rate using a PLSNSR1 pulse sensor. NodeMCU converts the input heart rate to beats per minute and calories burned. The output data is sent back to the Blynk app to be shown in the user interface.
In the user interface, the BPM (Beats per minute) will be updated every second, and the number of calories burned will be updated every 10 seconds, allowing the user to track the intensity of his or her exercise regimen.
Upon completion of the session, the user must power off the system via the Blynk app interface. Now, an email including the number of calories burned and the duration of the session will be sent to the user’s email in order to help them maintain a log of calories burned.
Iot Fitness Band Features:
- Determines the average heart rate in BPM (bits per minute).
- Calculates and updates the total number of calories burned in the cloud every 10 seconds.
- Through an IoT application or smartphone, the user can control when the fitness band activity begins and ends.
- Sends the user an email with their average heart rate (BPM) and calories burned.
- A blinking LED indication that corresponds to the user’s heart rate.
Circuit Diagram:
User interface ( Blynk App ):
The screenshot displayed above depicts the user interface of the Blynk app UI utilised for this Fitness band project. Blynk is a program that provides embedded projects with cloud capability. It allows users to create their own User interface based on their project’s requirements.
The application takes the below three inputs
- Sex
- Age
- Weight
After receiving valid inputs, the system can be powered on. The ON or OFF button serves as the band’s on/off switch. When the user presses this button, the above three parameters will be transmitted to NodeMCU through the cloud, allowing calories to be calculated. When invalid data is entered into the Age and weight fields, i.e. zero, the fitness tracker will remain in the OFF state.
After processing sensor data, the NodeMCU will update app interface variables such as BPM (updated every second) and Calories burned (once in 10 seconds). This will aid users in real-time calorie tracking and assessing the intensity of their fitness regimen.
The user can turn OFF the device once the fitness session is complete. The user will receive an email containing their activity report when turning off the device.
Formulas:
The formulas necessary to convert heart rate to calories burned are as follows: –
For male
Calories burn = ((-55.0969 + (0.6309*HR) + (0.1988*W) + (0.2017*A))/4.184 )*60*T
For Female
Calories burn = ((-20.4022+(0.4472*HR) – (0.1263*W) + (0.074*A))/4.184)*60*T
Where
HR – Heartbeat Rate (in BPM)
W – Weight (in Kilograms)
A – Age(in years)
T – Duration time of exercise(in hours)
Prototype:
Connecting fitness band to IOT cloud:
Add the SSID and password for your smartphone to the code and submit to NodeMCU. Utilize the Authorization token generated by your Blynk application in the code. Wait for NodeMCU to establish a connection with your phone. Once the devices have been paired, the NodeMCU will await the user’s Device ON command.
BPM and calories burned will be calculated and updated until NodeMCU gets the Device Off instruction. If you wish to use a different smartphone to pair with a fitness band, you must update the code by changing the SSID and password of the target device.
Algorithm:
- Wait for the Blynk app to connect.
- Read the input from the Blynk user interface, including the user’s age, weight, and gender.
- Wait until the Device ON button is activated, and if it is, proceed to step 6.
- If the ON button is activated, the current system time should be stored in a variable.
- Convert the sensor analog input data (heartbeat) to Beats per minute ( BPM ). Transfer the BPM to the Blynk UI.
- Calculate the number of calories burned every ten seconds. Upload burned calories to the Blynk UI.
- Repeat until the ON button is deactivated (OFF).
- Display the final burned calories and heart rate in the Blynk UI.
Code:
Include the following header files in your Arduino IDE: ESP8266WiFi.h and BlynkSimpleEssentials.h.You can find the Fitness band – Github repository here.
// Include header files #define BLYNK_PRINT Serial #include<ESP8266WiFi.h> #include <BlynkSimpleEsp8266.h> // Auth token generated by Blynk char auth[] = “gTJf3EcGRGacgcW24I-OWcmj70OlwjZ2”; // Your WiFi credentials. // Set password to “” for open networks. char ssid[] = “Your SSID”;     //Replace with your SSID char pass[] = “Your Password”;   //Replace with your password // Extenal LED int led_pin = D6; // Variables required for calibrations const int postingInterval = 10 * 1000; int rate[10];                    unsigned long sampleCounter = 0; unsigned long lastBeatTime = 0;  unsigned long lastTime = 0, N; int BPM = 0; int IBI = 0; int P = 512; int T = 512; int thresh = 512;  int amp = 100;                   int Signal; boolean Pulse = false; boolean firstBeat = true;          boolean secondBeat = true; boolean QS = false;    //Variables required to calculations boolean time_noted=false; unsigned long starting_time=0;    //starting time of the device in millis second boolean mail_sent=false;    unsigned long average_bpm=0;     // stores average bpm unsigned long bpm_counted=0;     // stores number of times bpm is calculated boolean male=true; int weight=0; int age=0; int start_button=0; int does_mail_send =0; int heart_rate=0; float calorie_burn; float time_duration=0; float total_calorie_burn=0; // Setup block void setup() {   // put your setup code here, to run once:    Serial.begin(115200);    //Pinmodes    pinMode(A0,INPUT);    pinMode(led_pin,OUTPUT);    //Blynk setup    Blynk.begin(auth, ssid, pass); } void loop() {   // put your main code here, to run repeatedly:   Blynk.run();  // Blynk will run continuously   // here if block will execute when start button in the blynk is ON   if(start_button==1 && time_noted==true){         if(check_if_data_is_correct()==false) continue;  // checks if the user has entered age, gender and weight before turning on the device         //Serial.println(“BPM is Calculating”);     calculate_bpm();            if(millis()-starting_time>=10000){         calculate_calories_10sec(); // calculate calories burnt for every 10 minutes     }   }   // else if block will get executed when the starts button gets off   else if(mail_sent==true){     calculate_calories_10sec();     send_email();     mail_sent=false;     reset_bpm();  // reset the variables when device gets OFF   } } // checks if the user has entered age, gender and weight before turning on the device bool check_if_data_is_correct(){   if(weight<=0 || age<=0){     Blynk.virtualWrite(V9, LOW);     start_button=0;     time_noted=false;     reset_bpm();    return false;   }   return true; } // This function calculates calories burnt in every 10 seconds and update it into blynk void calculate_calories_10sec(){   starting_time=millis();   heart_rate=average_bpm/bpm_counted;   if(male==true){     Serial.println(“Male”);     calorie_burn=(float)( ((-55.0969+(0.6309*heart_rate)+(0.1988*weight)+(0.2017*age))/4.184)*60*0.00277778);   }   else{     Serial.println(“Female”);     calorie_burn=(float)( ((-20.4022+(0.4472*heart_rate)-(0.1263*weight)+(0.074*age))/4.184)*60*0.00277778);   }   total_calorie_burn+=calorie_burn;   Serial.println(weight);   Serial.println(age);   Serial.println(average_bpm);   Serial.println(bpm_counted);   Serial.println(calorie_burn);   Serial.println(time_duration);   Serial.println(starting_time);   Blynk.virtualWrite(V7,total_calorie_burn); } // this function is used to send email to the user about his status when the device gets off void send_email() {   if(does_mail_send == 0){     String msg=”Hi Nihar Gupta. Your average heart beat is:”+(String)heart_rate+”. Your “+(String)calorie_burn+”calories are burned during the time of”+(String)time_duration+”Hrs”;     Blynk.email(“[email protected]”, “Heart Beat Notification”, msg); //Replace with your email     does_mail_send = 1;   } } // Reset the variables when the device is get off void reset_bpm(){   average_bpm=0;   bpm_counted=0;   total_calorie_burn=0;   Blynk.virtualWrite(V7,0);   Blynk.virtualWrite(V1,0); } // This function will take care to initilize BPM void calculate_bpm(){   if (QS == true) {    Serial.println(“BPM: “+ String(BPM));    Blynk.virtualWrite(V1,BPM);    average_bpm+=BPM;    bpm_counted++;    QS = false;    } else if (millis() >= (lastTime + 2)) {      readPulse();      lastTime = millis();    }         } // This function will read the analog data and calibrate the data into BPM void readPulse() {   Signal = analogRead(A0);         analogWrite(led_pin,Signal);      sampleCounter += 2;                             int N = sampleCounter – lastBeatTime;     detectSetHighLow();   if (N > 250) {      if ( (Signal > thresh) && (Pulse == false) && (N > (IBI / 5) * 3) )       pulseDetected();   }   if (Signal < thresh && Pulse == true) {      Pulse = false;     amp = P – T;     thresh = amp / 2 + T;      P = thresh;     T = thresh;   }   if (N > 2500) {     thresh = 512;     P = 512;     T = 512;     lastBeatTime = sampleCounter;     firstBeat = true;                secondBeat = true;             } } void detectSetHighLow() {   if (Signal < thresh && N > (IBI / 5) * 3) {     if (Signal < T) {                             T = Signal;                             }   }   if (Signal > thresh && Signal > P) {        P = Signal;                             }                                       } // This calibration is when a pulse is detected void pulseDetected() {   Pulse = true;                             IBI = sampleCounter – lastBeatTime;       lastBeatTime = sampleCounter;             if (firstBeat) {                           firstBeat = false;                     return;                              }   if (secondBeat) {                        secondBeat = false;                    for (int i = 0; i <= 9; i++) {         rate[i] = IBI;     }   }   word runningTotal = 0;                     for (int i = 0; i <= 8; i++) {              rate[i] = rate[i + 1];                runningTotal += rate[i];            }   rate[9] = IBI;                        runningTotal += rate[9];              runningTotal /= 10;                   BPM = 60000 / runningTotal;           QS = true; }                           // To know Start button in blynk is ON/OFF BLYNK_WRITE(V9) {   start_button = param.asInt();   if(start_button==1 && time_noted==false){     starting_time=millis();     mail_sent=false;     does_mail_send=0;     time_noted = true;   }   else if(start_button==0 && time_noted==true){     mail_sent = true;     time_noted=false;   } } // Update gender when it is changed BLYNK_WRITE(V2){   switch(param.asInt()){     case 1:{ male=true;              break;            }     case 2:{male=false;             break;                 }   } } // update weight when it is changed in Blynk BLYNK_WRITE(V3){   weight = param.asInt(); } // update age when it is changed in Blynk BLYNK_WRITE(V4){   age=param.asInt(); } |
Conclusion
I hope all of you have understood how to design IoT based Fitness band using NodeMCU and Pulse sensor. We MATHA ELECTRONICS will be back soon with more informative blogs soon.